前缀、文档修改记录
20250509 初始化编辑此文章,完稿
章节1、需求描述
Debian 12系统下,安装chrome浏览器,配套安装chrome driver,以便用程序来驱动Chrome浏览器
章节2、操作步骤
2.1、安装必要的工具
root@server:~# apt update root@server:~# apt install wget curl unzip
2.2、下载Chrome浏览器
root@server:~# mkdir -p /root/src root@server:~# cd /root/src root@server:~# wget https://dl.google.com/linux/direct/google-chrome-stable_current_amd64.deb // 从 Google 官方下载安装包
2.3、安装Chrome浏览器
使用 dpkg 安装,如果有依赖问题,再用 apt 修复即可
root@server:~# cd /root/src/ root@server:~# dpkg -i google-chrome-stable_current_amd64.deb // 本次安装到的版本是136.0.7103.92-1 Tag-2025-5-9 root@server:~# apt --fix-broken install -y // 执行路径 /usr/bin/google-chrome
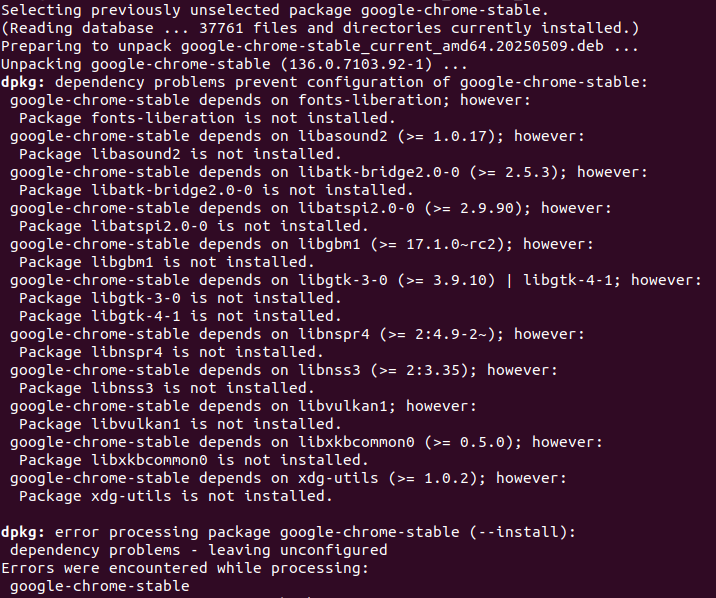
确认版本信息
root@server:~# /usr/bin/google-chrome -version Google Chrome 136.0.7103.92 // Tag-2025-5-9 输出版本号,说明安装成功
2.4、下载和安装 ChromeDriver
ChromeDriver 版本需要和 Chrome 浏览器版本对应,所以,刚刚安装的Chrome版本务必记录下来。
低于115的版本的官方地址 https://chromedriver.chromium.org/downloads
https://developer.chrome.com/docs/chromedriver/downloads
图/官方提示:

高于115或者更新版本的官方地址 https://googlechromelabs.github.io/chrome-for-testing/
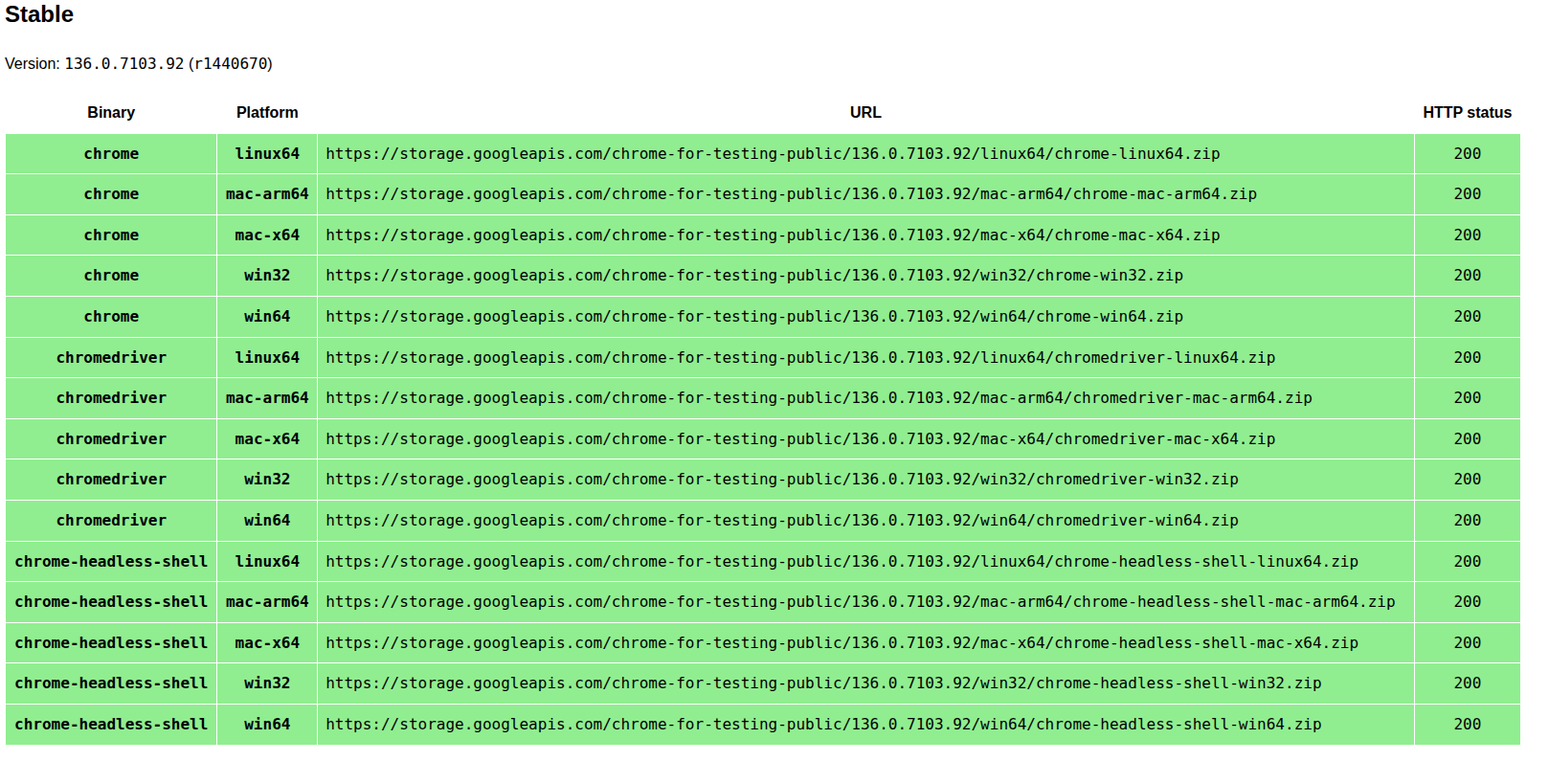
下载其中chromedriver的linux64版本即可,https://storage.googleapis.com/chrome-for-testing-public/136.0.7103.92/linux64/chromedriver-linux64.zip
root@server:~# cd /root/src/ root@server:~# wget https://storage.googleapis.com/chrome-for-testing-public/136.0.7103.92/linux64/chromedriver-linux64.zip // 版本编号要跟Chrome浏览器的一致 root@server:~# unzip chromedriver-linux64.zip Archive: chromedriver-linux64.zip inflating: chromedriver-linux64/LICENSE.chromedriver inflating: chromedriver-linux64/THIRD_PARTY_NOTICES.chromedriver inflating: chromedriver-linux64/chromedriver root@server:~# root@server:~# mv chromedriver-linux64/chromedriver /usr/bin/chromedriver root@server:~# chmod +x /usr/bin/chromedriver // 挪目录,赋权限 root@server:~# chromedriver --version // 确认版本号,显示就是正常的,务必跟Chrome版本一致
章节3、测试代码
#!/usr/bin/python3 # -*- coding:utf-8 -*- # by Dasmz # 2025-5-9 # import time import random from datetime import datetime from selenium import webdriver from selenium.webdriver.common.by import By from selenium.webdriver.support.ui import WebDriverWait from selenium.webdriver.support import expected_conditions as EC CHROMEDRIVER_PATH = '/usr/bin/chromedriver' SCREENSHOT_PATH = '/var/www/html/ScreenShot.png' URL_LIST = ['URL1','URL2','URL3','URL4','URL5'...] def getNow(): now = datetime.now() return now.strftime("%Y-%m-%d %H:%M:%S") def create_driver(user_agent): chrome_options = webdriver.ChromeOptions() chrome_options.add_argument('--headless') # 无界面模式 chrome_options.add_argument("--window-size=1920x1080") # 窗口大小 chrome_options.add_argument('--disable-gpu') # 关闭GPU加速 chrome_options.add_argument("--mute-audio") # 关闭声音 chrome_options.add_argument("--no-sandbox") # 无沙箱 chrome_options.add_argument("--disable-dev-shm-usage") # 不使用/dev/shm共享内存目录 chrome_options.add_argument("--disable-extensions") # 关闭扩展程序 chrome_options.add_argument("--log-level=3") # 只显示致命错误FATAL chrome_options.add_argument('--disable-application-cache') # 关闭 Chrome 的应用缓存,包括页面缓存、离线缓存 chrome_options.add_argument('--incognito') # 隐身模式,每次打开网页都是新会话,不会用缓存、也不会保存 cookie chrome_options.add_argument('--disk-cache-size=0') # 磁盘缓存设为 0 字节 chrome_options.add_argument(f"--user-agent={user_agent}") # 禁止图片加载 # prefs = {"profile.managed_default_content_settings.images": 2} # chrome_options.add_experimental_option("prefs", prefs) driver = webdriver.Chrome(executable_path=CHROMEDRIVER_PATH, options=chrome_options) driver.set_window_size(1920, 1080) # 设置分辨率 (宽, 高) return driver def run_chrome(driver, url): try: driver.get(url) # 等待 body 元素加载完成 time.sleep(1.5) # 给浏览器的网页加载的时间 WebDriverWait(driver, 10).until(EC.presence_of_element_located((By.TAG_NAME, 'body'))) print(f"标题: {driver.title}") print(f"URL: {driver.current_url}") # print(f"页面源码长度: {len(driver.page_source)}") # 网页加载情况截图 driver.save_screenshot(SCREENSHOT_PATH) except Exception as e: print(f"出错: {e}") def go2web(driver): try: target_url = random.choice(URL_LIST) run_chrome(driver, target_url) except Exception as e: print(f"访问出错: {e}") if __name__ == '__main__': user_agent = 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/135.0.0.0 Safari/537.36' driver = create_driver(user_agent) try: for i in range(1, 40000000): print(f"\n\n第 {i} 次访问 {getNow()}") go2web(driver) # time.sleep(1) # 可调整等待时间 except KeyboardInterrupt: print("手动中断") finally: driver.quit()
附录1、视频操作演示
附录2、@Dasmz
博客内,所有教程为手打原创教程,如果技术教程对您有所帮助,欢迎打赏作者。技术层面,闻道有先后,如有疏漏、错误,欢迎指正。技术博客的内容,一般具有一定的环境依赖,具有一定的年代依赖,酌情参考其中的内容,请勿完全照搬照抄。
对于博客内已提及的专业知识,如果需要技术指导,欢迎联系我,仅需支付工时费
Twitter: Dasmz
Youtube: @DasmzStudio
Telegram: @Dasmz
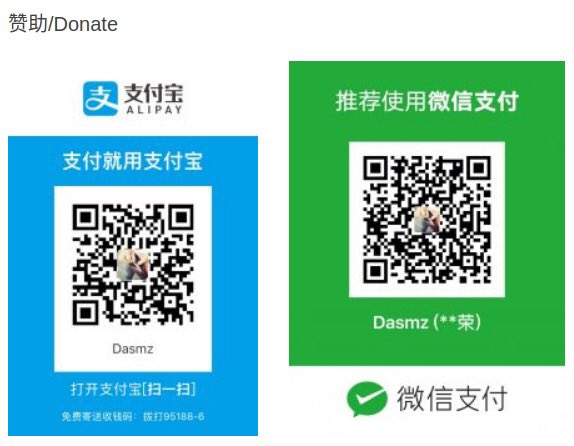